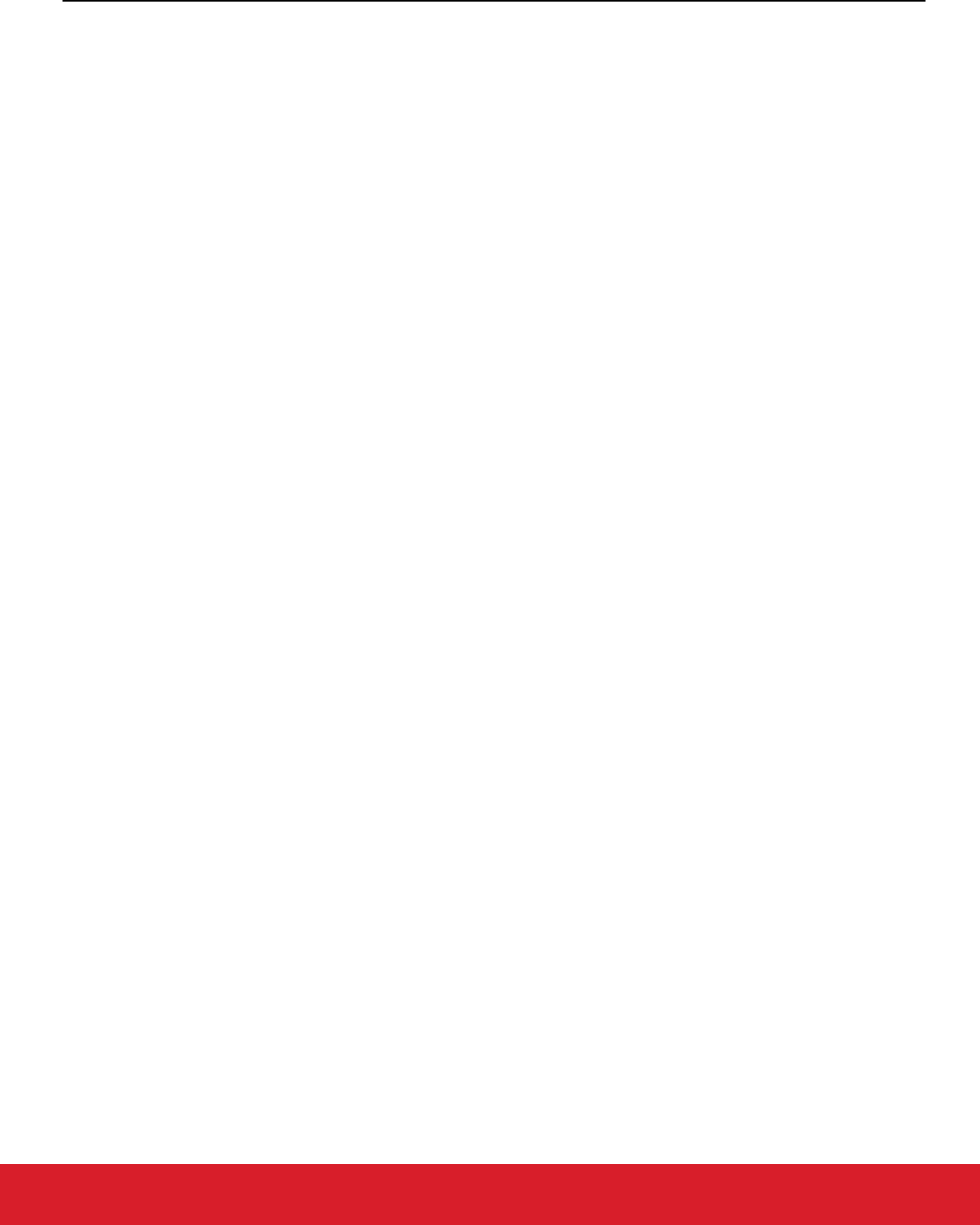
UG491: Zigbee Application Framework Developer's Guide for SDK 7.0 and Higher
Sleepy Devices
silabs.com | Building a more connected world. Rev. 0.5
silabs.com | Building a more connected world. Rev. 0.5 | 27
14.2.2 The LONG_POLL Interval
The LONG_POLL interval is the amount of time that an end device may wait before polling its parent when it is otherwise inactive. This
interval should, but is not required to, be shorter than the End Device Poll Timeout, which is the amount of time a parent device will wait
to hear from its child before removing it from its child tables. The default end device poll timeout for Zigbee devices is set to 256 minutes.
Note: The Zigbee protocol does not offer a standard way to timeout entries in a child table. In place of this, several heuristic mecha-
nisms exist for aging entries in a child table. For instance, if a parent hears a device that it thinks is its child interacting with
another parent or being represented by another parent, it may remove the entry from its child table. Silicon Labs has developed
a more deterministic mechanism for child aging called the End Device Poll Timeout. A parent expects that children will “check
in” with their parents within the end device poll timeout. If they do not, it assumes that they have gone away and removes them
from its child tables. The End Device Poll Timeout may be modified from its default value by configuring the Zigbee stack or
leaf components, depending on the local device type.
The end device does not get to configure the end device poll timeout on its parent and there is no agreed upon protocol for communi-
cating the End Device Poll Timeout value between parent and child. In place of this, Silicon Labs has configured an assumed end device
poll timeout on both parent and child.
Depending on its sleep characteristics and battery life considerations, the child may wish to sleep past the assumed end device poll
timeout. It is free to do this. However, if it does, it must repair the network connection by performing a network rejoin operation before
interacting with the network again. Generally, a device that is likely to do this should check the state of the network when it wakes up to
see if any repair is necessary before sending data. A sleepy device should never wake and assume that its parent is still there, unless
it knows for certain that its parent is configured with a mutually agreed upon End Device Poll Timeout that it is obeying. For more
information on the end device poll timeout see the configuration header file located at stack/include/ember-configuration-defaults.h.
14.2.2.1 Setting at Compile Time
The LONG_POLL interval is configurable in one-second increments and may be modified at compile-time by configuring the End device
support component. This sets the define EMBER_AF_PLUGIN_END_DEVICE_SUPPORT_LONG_POLL_INTERVAL_SECONDS in
code.
14.2.2.2 Setting at Run Time
You can modify the _LONG_POLL at runtime using the callbacks
emberAfSetLongPollIntervalMsCallback or emberAf-
SetLongPollIntervalQsCallback
.
14.2.3 Setting Values for the SHORT_POLL and LONG_POLL Intervals
The SHORT_POLL Interval should be less than the Indirect Transmission Timeout of the parent to prevent lost data/ACKs (< 7.8 sec-
onds). The LONG_POLL Interval should be less than the End Device Poll Timeout of the parent (assuming the parent implements an
End Device Poll Timeout) to prevent the parent from aging out the end device due to inactivity. By default, the Zigbee stack ships with
a 256-minute End Device Poll Timeout. The manufacturer can change the End Device Poll Timeout as they wish. There is no standard
way for routers to report their chosen End Device Poll Timeout to their children and it is not required for routers to implement child aging
in the Zigbee specification. As a result, if a device implements a LONG_POLL_INTERVAL that is longer than 256 minutes, Silicon Labs
recommends that the device check its network status before spontaneously sending messages through its parent. You want the device
to make sure that the connection to the parent is up before it sends a message. If the network is not up, the device should perform a
network rejoin to make sure that it has a parent before sending any message out over the air.
14.2.4 Forcing Fast Polling
Fast Polling is the state during which the stack actively polls its parent device faster than the 7.68 second child message timeout interval.
The Zigbee Application Framework polls at the rate defined by the SHORT_POLL interval when it is in this mode. The Zigbee Application
Framework automatically keeps the stack in the fast poll mode during the sending and ACKing of an APS message. When a device
sends a message that is part of a series of application-level request/responses—as is the case in Smart Energy Registration—it must
keep the device in fast poll mode until the entire transaction is completed.
The Zigbee Application Framework can ensure that the application stays in short poll mode for as long as the application requires by
setting a flag in the
emberAfCurrentAppTasks mask. To do this, create your own flag for the emberAfCurrentAppTasks